In Python, functions can be called multiple times from different parts of the program, making the code more modular and easier to maintain. They are defined using the def keyword, followed by the function name and a set of parentheses containing the function parameters.
The syntax of a Python function is straightforward. It starts with the def keyword, followed by the function name, and a set of parentheses that may contain zero or more parameters. The function body is indented and must contain at least one statement.
Defining a Python function
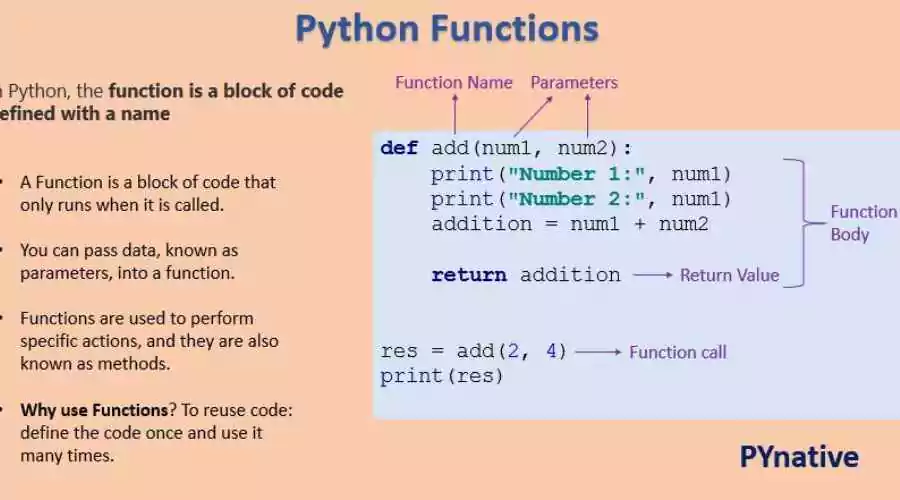
It is a code that can be reused to carry out a particular operation and is known as a function. It takes input, performs operations on it, and returns output. In Python, functions are defined using the “def” keyword, followed by the function name, parentheses, and colon. The syntax for defining a Python function is as follows:
def function_name(arguments):
statement(s)
return expression
Let’s break down the syntax and understand each element.
“def”: It is a keyword that tells Python we are defining a function.
“function_name”: It is the name of the function, which should be descriptive and meaningful.
“arguments”: These are the inputs or parameters passed to the function. We are free to choose any number of characteristics.
“colon”: It signifies the end of the function header and the start of the function body.
“statement(s)”: It is a set of statements that perform some operations on the inputs or variables.
“return”: It specifies the output of the function.
Once we define a function, we can call it from anywhere in our code by passing the required arguments.
Python function parameters
Python functions can have multiple parameters, and we can pass them in two ways – positional and keyword arguments. Positional arguments are passed in the order they appear in the function definition, whereas keyword arguments are passed with the parameter name followed by a colon and the value. Let’s see an example of both types of arguments.
def add_numbers(x, y):
sum = x + y
return sum
# Positional Arguments
result = add_numbers(3, 5)
print(result) # Output: 8
# Keyword Arguments
result = add_numbers(x=3, y=5)
print(result) # Output: 8
In the above example, we defined a function “add_numbers” that takes two parameters, “x” and “y,” and returns their sum. We called this function twice – once with positional arguments and once with keyword arguments. Both ways resulted in the same output.
How to Call a Python function
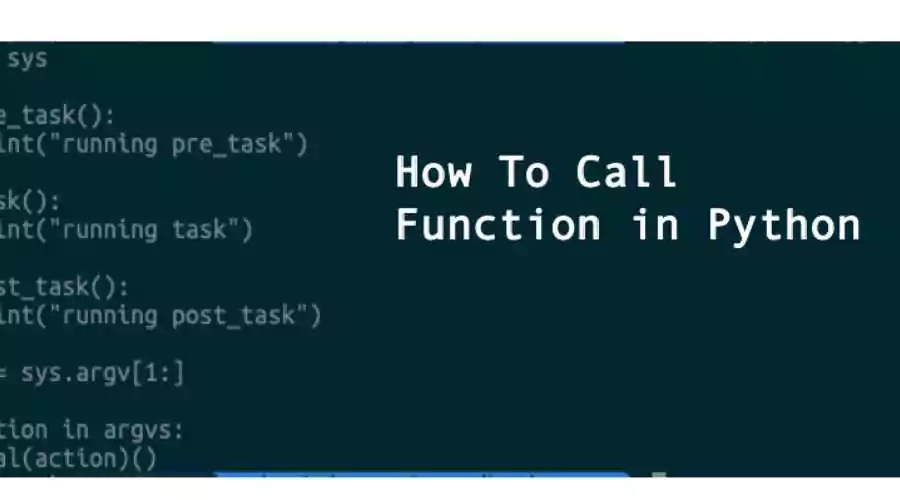
A function can be created in Python by first typing the def keyword, then typing the function’s name, and finally typing parentheses. The Python code that makes up the block of code that specifies the function is indented and can be anything that’s legal in Python. The following is an illustration of a straightforward function that accepts two parameters and outputs the sum of those parameters:
def add_numbers(a, b):
return a + b
To call this function, we need to provide the values for the a and b parameters. We can do this by passing the values as arguments to the function, as shown below:
result = add_numbers(5, 10)
print(result) # Output: 15
In this example, we are calling the add_numbers function with the arguments 5 and 10. The function returns the sum of these two values, which is stored in the result variable. We then print the value of the result, which is 15.
Positional parameters in Python functions
In Python functions, parameters can be either positional or keyword. Positional parameters are the most common type of parameters, and they are defined in the function header. When calling a function with positional parameters, the values are passed in the order that they appear in the function header.
Here is an example of a function with two positional parameters:
def greet(name, message):
print(f”{message}, {name}!”)
To call this function, we need to provide the values for the name and message parameters in the order that they appear in the function definition:
greet(“Alice”, “Hello”) # Output: Hello, Alice!
In this example, we are calling the greet function with the arguments “Alice” and “Hello”. The function prints the message “Hello, Alice!” to the console.
Keyword parameters in Python functions
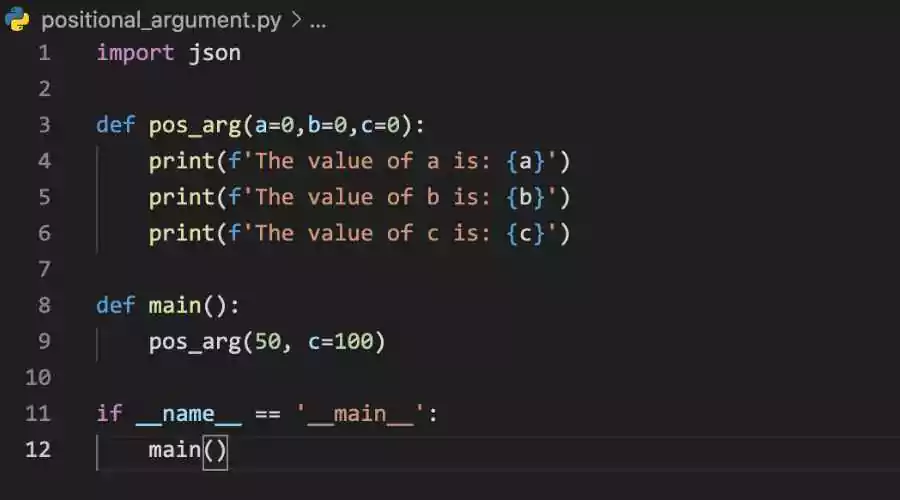
Keyword parameters are another type of parameter in Python functions. They are defined using the syntax parameter_name=default_value in the function header. When calling a function with keyword parameters, we can pass the values in any order and specify the parameter name.
Here is an example of a function with two keyword parameters:
def calculate_discount(price, discount_rate=0.1):
discount_amount = price * discount_rate
discounted_price = price – discount_amount
return discounted_price
In this example, the calculate_discount function takes two parameters: price and discount_rate. The discount_rate parameter has a default value of 0.1, which means that if we don’t pass a value for this parameter, it will default to 0.1.
We can call this function with the price parameter and override the default value of discount_rate using the keyword syntax:
price = 100
discounted_price = calculate_discount(price, discount_rate=0.2)
print(discounted_price) # Output: 80.0
In this example, we are calling the calculate_discount function with the price parameter set to 100 and the discount_rate parameter set to 0.2. The function returns the discounted price, which is 80.0.
Conclusion
Python is a versatile programming language with a wide range of functionalities. One of the most powerful features of Python is the ability to define and use functions. Functions in Python are self-contained blocks of code that can be reused throughout a program. There are several types of Python functions, each with its unique characteristics. For more information, visit SaveWithNerds.
FAQs